GraphQL: The future of APIs ๐
GraphQL vs. REST: Discover the advantages and disadvantages of the modern query language for APIs! ๐
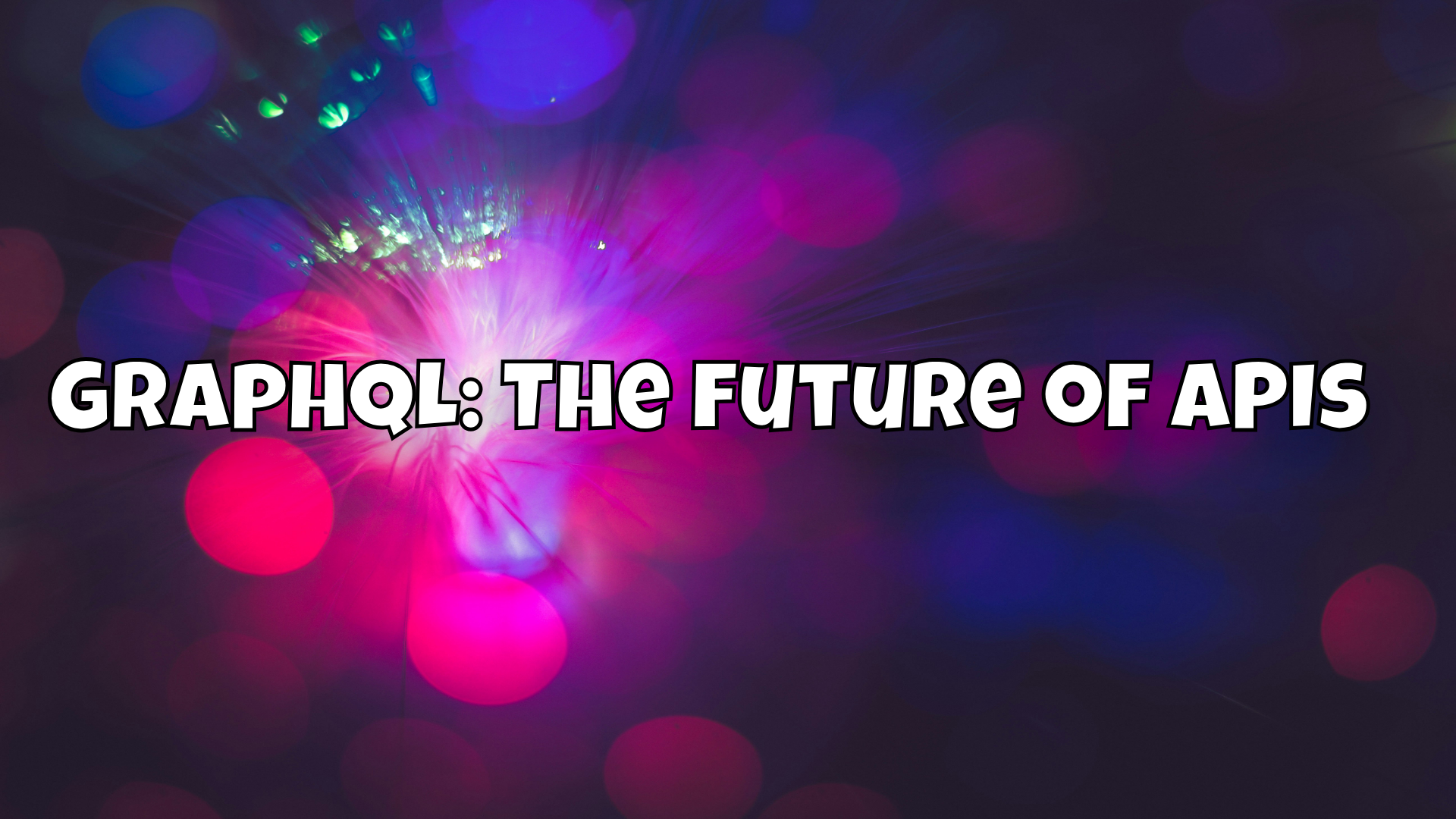
Imagine if you could customize an API to deliver exactly the data you need, no more and no less. That's the promise of GraphQL. While RESTful APIs have been the standard for years, GraphQL offers a more modern and flexible alternative. In this article, we take a detailed look at GraphQL and compare the pros and cons with RESTful APIs. I have already explained what exactly REST APIs are in a previous article ๐
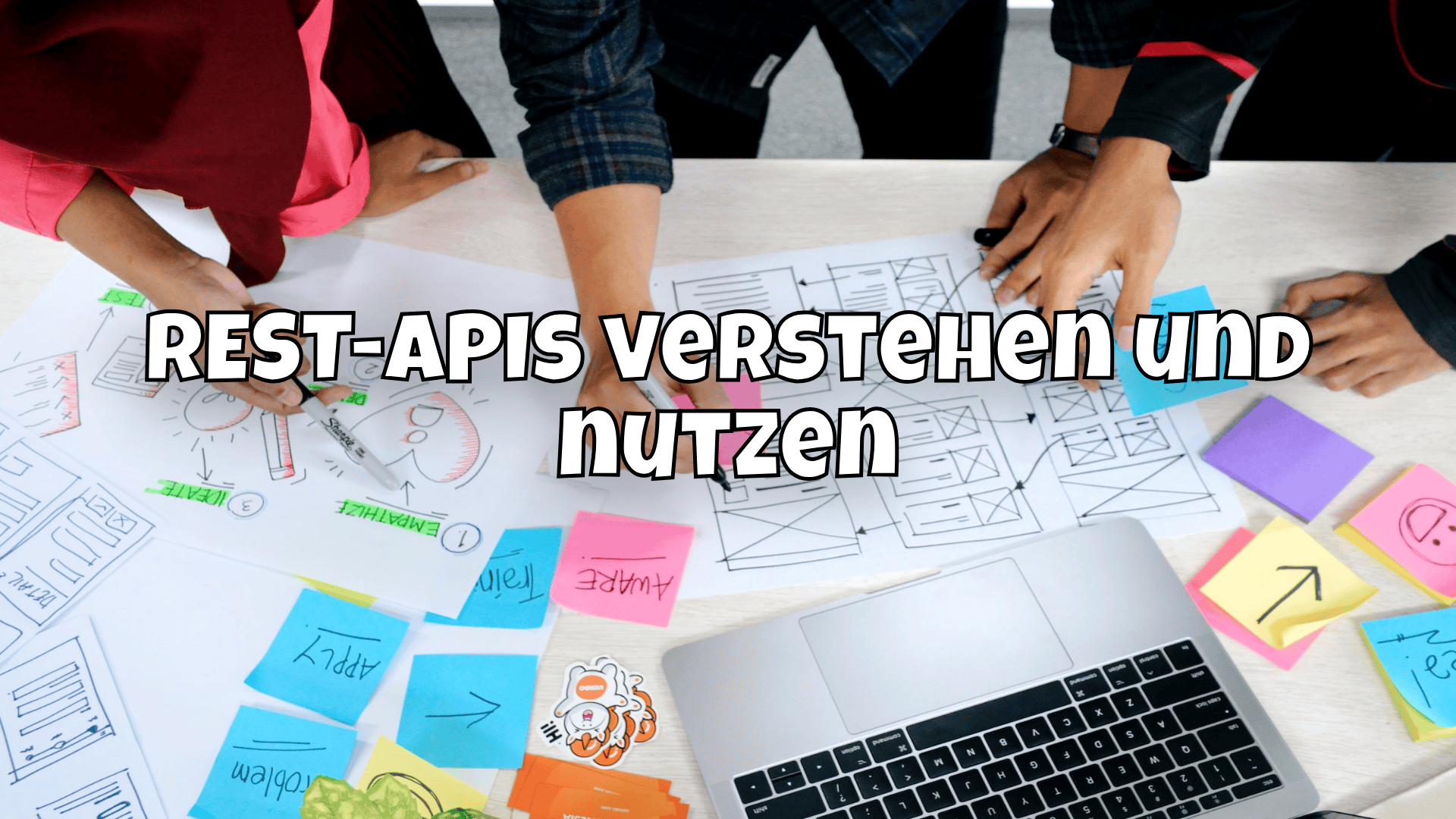
What is GraphQL? ๐ง
GraphQL is a query language for APIs and a runtime environment for fulfilling these queries with your existing data. Facebook developed it in 2015 to address the challenges of REST and enable more efficient data querying.
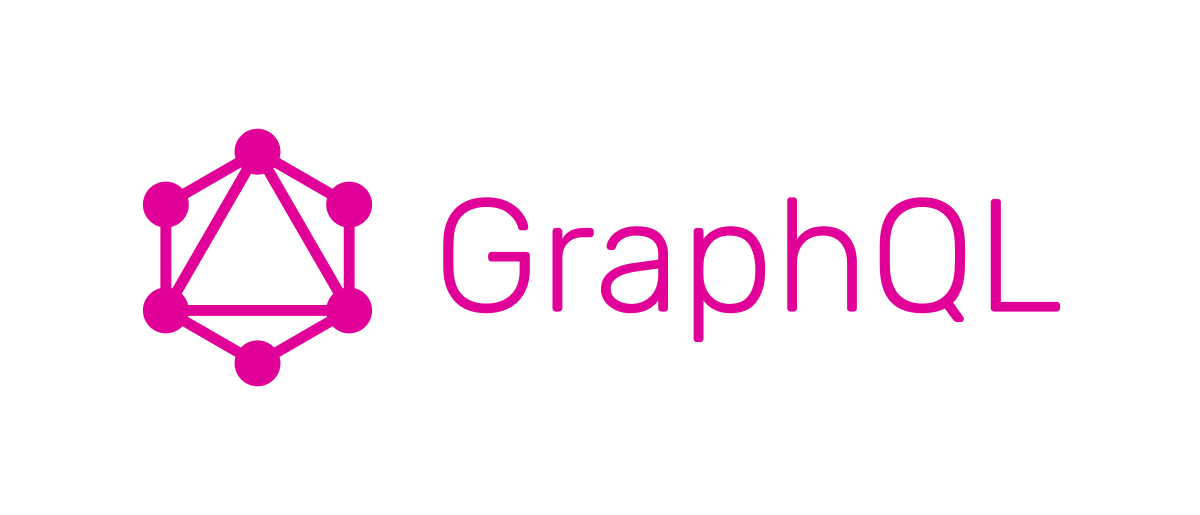
Functionality of GraphQL ๐ ๏ธ
With GraphQL, the client makes a query to the server and specifies exactly what data is required. The server responds with the desired data in exactly the requested structure. This is in contrast to REST, where the server specifies the structure of the response.
query {
user(id: "1") {
name
email
}
}
The example above shows a simple GraphQL query that requests the name and email address of a user with the ID 1.
Advantages of GraphQL ๐
Efficient data queries ๐
GraphQL allows the client to specify exactly the data required. This reduces the amount of data transferred and improves efficiency, especially for mobile applications with limited bandwidth.
Flexibility and adaptability ๐คนโโ๏ธ
Because the client determines the structure of the response, the API can be easily adapted to different requirements without the need for changes on the server side.
Strong typing ๐
GraphQL uses a strongly typed schema that allows queries to be validated in advance and detailed error messages to be generated. This improves the reliability and maintainability of the API.
Real-time updates with subscriptions ๐ก
GraphQL supports subscriptions, which make it possible to receive real-time updates of data. This is particularly useful for applications that require up-to-date information, such as chat applications or dashboards.
Disadvantages of GraphQL โ
Complexity of implementation ๐งฉ
GraphQL can be more complex to implement than REST, especially for developers who are not familiar with the technology. It requires a thorough understanding of the GraphQL schema and query language.
Security risks ๐ก๏ธ
Since the client defines the queries, there is a higher risk of inefficient queries that can lead to server overload. It is important to implement mechanisms to limit and monitor queries.
Caching challenges ๐๏ธ
Caching GraphQL queries is less straightforward than with REST, where resources can be uniquely identified and cached. It requires additional strategies and tools to implement efficient caching.
Comparison: GraphQL vs. REST ๐ฅ
Data query
- GraphQL: The client can specify exactly what data is required.
- REST: The server determines the structure of the response, which often leads to overfetching or underfetching.
Flexibility
- GraphQL: Very flexible, as the client defines the queries.
- REST: Less flexible, as the API endpoints and their responses are fixed.
Performance
- GraphQL: More efficient, as only the required data is transferred.
- REST: Can be inefficient if more data than necessary is transferred.
Real-time updates
- GraphQL: Supported by subscriptions.
- REST: Must be implemented using additional mechanisms such as WebSockets.
Practical examples with JavaScript ๐
Let's take a look at some practical examples to illustrate the difference between REST and GraphQL.
Example 1: Retrieve user information
REST
With a RESTful API, you would normally call multiple endpoints to get all the data you need.
/// Retrieve user information
fetch('https://api.example.com/users/1')
.then(response => response.json())
.then(user => {
console.log(user.name);
console.log(user.email);
// Retrieve further details
fetch(`https://api.example.com/users/1/posts`)
.then(response => response.json())
.then(posts => console.log(posts));
});
Here, the user is retrieved first and then the user's posts are retrieved in a second call. This leads to multiple network calls and potentially inefficient data transfer.
GraphQL
With GraphQL, all required data can be retrieved in a single query.
const query = `
query {
user(id: "1") {
name
email
posts {
title
content
}
}
}
`;
fetch('https://api.example.com/graphql', {
method: 'POST',
headers: {
'Content-Type': 'application/json'
},
body: JSON.stringify({ query })
})
.then(response => response.json())
.then(data => {
const user = data.data.user;
console.log(user.name);
console.log(user.email);
console.log(user.posts);
});
In this example, a single query is used to retrieve both the user information and the user's posts, resulting in a more efficient data transfer.
Example 2: Updating a user
REST
In a RESTful API, a user is updated via a PUT or PATCH call.
fetch('https://api.example.com/users/1', {
method: 'PUT',
headers: {
'Content-Type': 'application/json'
},
body: JSON.stringify({
name: 'New name',
email: '[email protected]'
})
})
.then(response => response.json())
.then(user => console.log(user));
GraphQL
In GraphQL, this is achieved by a mutation.
const mutation = `
mutation {
updateUser(id: "1", input: {
name: "New name",
email: "[email protected]"
}) {
name
email
}
}
`;
fetch('https://api.example.com/graphql', {
method: 'POST',
headers: {
'Content-Type': 'application/json'
},
body: JSON.stringify({ query: mutation })
})
.then(response => response.json())
.then(data => {
const user = data.data.updateUser;
console.log(user.name);
console.log(user.email);
});
Here, the example shows how a mutation is used to update the user information and at the same time get back the changed data.
Set up GraphQL server with Node.js ๐
To create a GraphQL server with Node.js, we need the following tools and libraries:
- Node.js
- Express.js

- Apollo Server (GraphQL server library)
Step 1: Set up a project
Create a new directory and initialize a Node.js project:
mkdir graphql-server
cd graphql-server
npm init -y
Install the required dependencies:
npm install express apollo-server-express graphql
Step 2: Define GraphQL schema and resolvers
Create a file schema.js
and define the GraphQL schema and the resolvers:
const { gql } = require('apollo-server-express');
// Define the GraphQL schema
const typeDefs = gql`
type User {
id: ID!
name: String!
email: String!
}
type Query {
user(id: ID!): User
}
type Mutation {
updateUser(id: ID!, name: String!, email: String!): User
}
`;
// Example data
const users = [
{ id: '1', name: 'Max Mustermann', email: '[email protected]' },
];
// Define the resolvers
const resolvers = {
Query: {
user: (parent, args) => users.find(user => user.id === args.id),
},
Mutation: {
updateUser: (parent, args) => {
const user = users.find(user => user.id === args.id);
if (user) {
user.name = args.name;
user.email = args.email;
}
return user;
},
},
};
module.exports = { typeDefs, resolvers };
Step 3: Set up GraphQL server
Create a file index.js
and set up the GraphQL server:
const express = require('express');
const { ApolloServer } = require('apollo-server-express');
const { typeDefs, resolvers } = require('./schema');
const app = express();
const server = new ApolloServer({ typeDefs, resolvers });
server.applyMiddleware({ app });
app.listen({ port: 4000 }, () =>
console.log(`๐ Server ready at http://localhost:4000${server.graphqlPath}`)
);
Step 4: Start the server
Start the server:
node index.js
Now your GraphQL server is running at http://localhost:4000/graphql
. You can test the server by opening the URL in your browser and executing queries in the Apollo Playground.
Conclusion ๐
GraphQL offers many advantages over RESTful APIs, especially in terms of flexibility and efficiency. However, it also brings with it additional complexity and security challenges. For developers willing to take on these challenges, GraphQL can be a powerful and forward-looking technology.
If you have any questions or comments about GraphQL, feel free to leave a comment or just drop by the forum.